Create Payments Using OPay Cashier
Throughout this page, you will learn how to create payments using our OPay Cashier plugin. In this page you will learn how to:
- Collect your client's order information.
- Trigger OPay Cashier API with your collected order information.
- Handling OPay Cashier API Response.
- Redirect your client to OPay Cashier Checkout Page.
- Handling OPay Cashier Checkout Page Returned Payment Processing Information.
Collect your Client's Order Information
Once your client's order is ready, you will need to present your own payment details form. This form should collect:
- Client Information: Name, Phone, E-mail, Shipping Address.
- Order Information: Product, Quantity, Unit Price, Order Amount.
Once you have collected the required information, pass them to your server so your application can trigger OPay Cashier Plugin.
OPay Cashier Create Payment
To get started with OPay Cashier, you need to call the create cashier payment API. In case you are still in development phase, you will need to call create cashier payment API using POST method at the following staging endpoint API point URL
https://testapi.opaycheckout.com/api/v1/international/cashier/create
Once you have a fully tested payment flow and you are ready for production, you should use the following production API endpoint URL instead
https://liveapi.opaycheckout.com/api/v1/international/cashier/create
Cashier Create Payment API HTTP POST Parameters
Your request should contain:- Header:
- Bearer PublicKey: Your OPay merchant account Public Key.
- merchant ID: Your OPay account merchant ID.
- Json object containing the payment information:
Authorization: Bearer {PublicKey}
MerchantId : 256612345678901
{
"country": "NG",
"reference": "983541354",
"amount": {
"total": 400,
"currency": "NGN"
},
"returnUrl": "https://your-return-url",
"callbackUrl": "https://your-call-back-url",
"cancelUrl": "https://your-cacel-url",
"displayName":"sub merchant name",
"expireAt":300,
"sn":"PE462xxxxxxxx",
"userInfo":{
"userEmail":"test@email.com",
"userId":"userid001",
"userMobile":"+23488889999",
"userName":"David"
},
"product":{
"description":"description",
"name":"name"
},
"payMethod":"BankCard"
}
Detailed description of the parameters that you need to incorporate into your POST request are given in the table below.
An example call of cashier create payment is given below.
class CashierCreateController
{
private $publickey;
private $merchantId;
private $url;
public function __construct() {
$this->merchantId = '256621051120756';
$this->publickey = 'OPAYPUB*************54133';
$this->url = 'https://testapi.opaycheckout.com/api/v1/international/cashier/create';
}
public function test(){
$data = [
'country' => 'NG',
'reference' => '9835413542121',
'amount' => [
"total"=> "400",
"currency"=> 'NGN',
],
'returnUrl' => 'https://your-return-url',
'callbackUrl'=> 'https://your-call-back-url',
'cancelUrl' => 'https://your-cacel-url',
'expireAt' => 30,
'sn' => 'PE462xxxxxxxx',
'userInfo' => [
"userEmail"=> 'xxx@xxx.com',
"userId"=> 'userid001',
"userMobile"=> '13056288895',
"userName"=> 'xxx',
],
'product' => [
"name"=> 'name',
"description"=> 'description'
],
'payMethod'=>'BankCard',
];
$data2 = (string) json_encode($data,JSON_UNESCAPED_SLASHES);
$header = ['Content-Type:application/json', 'Authorization:Bearer '. $this->publickey, 'MerchantId:'.$this->merchantId];
$response = $this->http_post($this->url, $header, json_encode($data));
$result = $response?$response:null;
return $result;
}
private function http_post ($url, $header, $data) {
if (!function_exists('curl_init')) {
throw new Exception('php not found curl', 500);
}
$ch = curl_init();
curl_setopt($ch, CURLOPT_TIMEOUT, 60);
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($ch, CURLOPT_HEADER, false);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
curl_setopt($ch, CURLOPT_HTTPHEADER, $header);
$response = curl_exec($ch);
$httpStatusCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
$error=curl_error($ch);
curl_close($ch);
if (200 != $httpStatusCode) {
print_r("invalid httpstatus:{$httpStatusCode} ,response:$response,detail_error:" . $error, $httpStatusCode);
}
return $response;
}
}
# importing the requests library
import requests
# CashierAPI Endpoint
URL = "https://testapi.opaycheckout.com/api/v1/international/cashier/create"
# defining a params dict for the parameters to be sent to the API
PaymentData = {
'country' : 'NG',
'reference' : '12312321324',
'amount': {
"total": '400',
"currency": 'NGN',
},
'payMethod': 'BankCard',
'product': {
"name": 'Iphone X',
"description": 'xxxxxxxxxxxxxxxxx',
},
'returnUrl' : 'your returnUrl',
'callbackUrl' : 'your callbackUrl',
'cancelUrl' : 'your cancelUrl',
'userClientIP' : '1.1.1.1',
'sn' : 'PE462xxxxxxxx',
'expireAt' : 30,
}
# Prepare Headers
headers = {
'Content-Type' : 'application/json',
'Accept' : 'application/json',
'Authorization': 'Bearer Public Key',
'MerchantId' : '256612345678901'
}
# sending post request and saving the response as response object
status_request = requests.post(url = URL, params = json.dumps(PaymentData) , headers=headers)
# extracting data in json format
status_response = status_request.json()
const request = require('request');
const formData = {
"amount": {
"currency": "NGN",
"total": 400
},
"callbackUrl": "https://your-call-back-url",
"cancelUrl": "https://your-cacel-url",
"country": "NG",
"expireAt":300,
"sn":"PE462xxxxxxxx",
"payMethod":"BankCard",
"product":{
"description":"description",
"name":"name",
},
"reference": "983541354914",
"returnUrl": "https://your-return-url",
"userInfo":{
"userEmail":"xxx@xxx.com",
"userId":"userid001",
"userMobile":"13056288895",
"userName":"xxx"
}
};
var publickey = "OPAYPUB*************54133"
request({
url: 'https://testapi.opaycheckout.com/api/v1/international/cashier/create',
method: 'POST',
headers: {
'Authorization': 'Bearer '+publickey,
'MerchantId': '256621051120756'
},
json: true,
body: formData
}, function (error, response, body) {
console.log('body: ')
console.log(body)
}
)
curl --location --request POST 'https://testapi.opaycheckout.com/api/v1/international/cashier/create' \
--header 'MerchantId: 256621051120756' \
--header 'Authorization: Bearer OPAYPUB*************54133' \
--header 'Content-Type: application/json' \
--data-raw '{
"amount": {
"currency": "NGN",
"total": 400
},
"callbackUrl": "https://your-call-back-url",
"cancelUrl": "https://your-cacel-url",
"country": "NG",
"expireAt":300,
"sn":"PE462xxxxxxxx",
"payMethod":"BankCard",
"product":{
"description":"description",
"name":"name"
},
"reference": "983541354912",
"returnUrl": "https://your-return-url",
"userInfo":{
"userEmail":"xxx@xxx.com",
"userId":"userid001",
"userMobile":"13056288895",
"userName":"xxx"
}
}'
import com.google.gson.Gson;
import org.apache.commons.codec.binary.Hex;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.TreeMap;
import java.util.UUID;
public class CashierCreate {
private static final String publicKey = "OPAYPUB*************54133";
private static final String endpoint = "https://testapi.opaycheckout.com";
private static final String merchantId = "256621051120756";
public static void main(String[] args) throws Exception {
String addr = endpoint + "/api/v1/international/cashier/create";
Gson gson = new Gson();
TreeMap order = new TreeMap<>();
order.put("country","NG");
order.put("reference", UUID.randomUUID().toString());
TreeMap amount = new TreeMap<>();
amount.put("currency","NGN");
amount.put("total",new Integer(400));
order.put("amount",amount);
order.put("returnUrl","https://your-return-url.com");
order.put("callbackUrl","https://your-call-back-url.com");
order.put("cancelUrl","https://your-cacel-url");
order.put("expireAt",new Integer(300));
order.put("sn", "PE462xxxxxxxx");
TreeMap userInfo = new TreeMap<>();
userInfo.put("userEmail","xxx@xxx.com");
userInfo.put("userId","userid001");
userInfo.put("userMobile","13056288895");
userInfo.put("userName","xxx");
order.put("userInfo",userInfo);
TreeMap product = new TreeMap<>();
product.put("name","name");
product.put("description","description");
order.put("product",product);
order.put("payMethod","BankCard");
String requestBody = gson.toJson(order);
System.out.println("--request:");
System.out.println(requestBody);
URL url = new URL(addr);
HttpURLConnection con = (HttpURLConnection)url.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json; utf-8");
con.setRequestProperty("Authorization", "Bearer "+publicKey);
con.setRequestProperty("MerchantId", merchantId);
con.setDoOutput(true);
OutputStream os = con.getOutputStream();
byte[] input = requestBody.getBytes(StandardCharsets.UTF_8);
os.write(input, 0, input.length);
BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(), StandardCharsets.UTF_8));
StringBuilder response = new StringBuilder();
String responseLine = null;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
System.out.println("--response:");
System.out.println(response.toString());
//close your stream and connection
}
public static String hmacSHA512(final String data, final String secureKey) throws Exception{
byte[] bytesKey = secureKey.getBytes();
final SecretKeySpec secretKey = new SecretKeySpec(bytesKey, "HmacSHA512");
Mac mac = Mac.getInstance("HmacSHA512");
mac.init(secretKey);
final byte[] macData = mac.doFinal(data.getBytes());
byte[] hex = new Hex().encode(macData);
return new String(hex, StandardCharsets.UTF_8);
}
}
using System;
using System.IO;
using System.Linq;
using System.Net;
using System.Text;
using Newtonsoft.Json;
namespace OPayRequest
{
public class Program
{
static void Main(string[] args)
{
PostJson("https://testapi.opaycheckout.com/api/v1/international/cashier/create", new opay_request
{
country = 'NG',
reference = '12312321324',
amount= {
"total"= '400',
"currency"= 'NGN',
},
'payMethod': 'BankCard',
product= {
"name"= 'Iphone X',
"description"= 'xxxxxxxxxxxxxxxxx',
},
returnUrl = 'your returnUrl',
callbackUrl = 'your callbackUrl',
cancelUrl = 'your cancelUrl',
userClientIP = '1.1.1.1',
sn = 'PE462xxxxxxxx',
expireAt = 30,
});
}
private static void PostJson(string uri, opay_request postParameters)
{
string postData = JsonConvert.SerializeObject(postParameters);
byte[] bytes = Encoding.UTF8.GetBytes(postData);
var httpWebRequest = (HttpWebRequest) WebRequest.Create(uri);
httpWebRequest.Method = "POST";
httpWebRequest.ContentLength = bytes.Length;
httpWebRequest.ContentType = "text/json";
using (Stream requestStream = httpWebRequest.GetRequestStream())
{
requestStream.Write(bytes, 0, bytes.Count());
}
var httpWebResponse = (HttpWebResponse) httpWebRequest.GetResponse();
if (httpWebResponse.StatusCode != HttpStatusCode.OK)
{
string message = String.Format("GET failed. Received HTTP {0}", httpWebResponse.StatusCode);
throw new ApplicationException(message);
}
}
}
public class opay_request
{
public string reference { get; set; }
public string payMethod { get; set; }
public string returnUrl { get; set; }
public string callbackUrl { get; set; }
public string cancelUrl {get; set;}
public string userClientIP { get; set; }
public string sn { get; set; }
public string expireAt { get; set; }
public product product;
public amount amount;
}
public class amount
{
public string total { get; set; }
public string currency { get; set; }
}
public class product
{
public string name { get; set; }
public string description { get; set; }
}
}
Header must contain the "Signature" and "MerchantId"
Signature is calculated using the concatenation of the PublicKey and Bearer prefix:
Bearer {PublicKey}
OPay Cashier Response
Sample Response
the parameters contained in the response received whenever you call OPay Cashier Create API as a JSON Object.
Sample OPay Cashier Create API Response
{
"code": "00000",
"message": "SUCCESSFUL",
"data": {
"reference": "9835146212",
"orderNo": "211009140896553163",
"cashierUrl": "https://sandboxcashier.opaycheckout.com/apiCashier/redirect/payment/checkout?orderToken=TOKEN.546f3b0405b447be8198691999678765",
"status": "INITIAL",
"amount": {
"total": 400,
"currency": "NGN"
},
"vat": {
"total": 0,
"currency": "NGN"
}
}
}
Response Parameters Description
Parameter | type | Description | example | |
---|---|---|---|---|
reference | String |
Unique merchant payment order number. | 937102167 | |
orderNo | String |
Unique Opay payment order number. | 211009140896593010 | |
cashierUrl | String |
OPay Cashier URl generated for your client's current transaction. |
https://sandboxcashier.opaycheckout.com/apiCashier/redirect/payment/checkout?orderToken=TOKEN.546f3b0405b447be8198691999f56788 |
|
status | enum |
[INITIAL, PENDING, SUCCESS, FAIL, CLOSE] | SUCCESS | |
amount | ||||
total | Integer |
amount(cent unit). | 400 | |
currency | String |
currency type.See full list here | NGN | |
vat | ||||
total | Integer |
amount(cent unit). | 10 | |
currency | String |
currency type.See full list here | NGN |
Redirect Client to Cashier URL
Once you received the response of the cashier create payment API, your next action is to redirect your
client to the cashierUrl
received within the API response. Once you redirect your client, the cashier
page will be ready for your client to complete the payment.
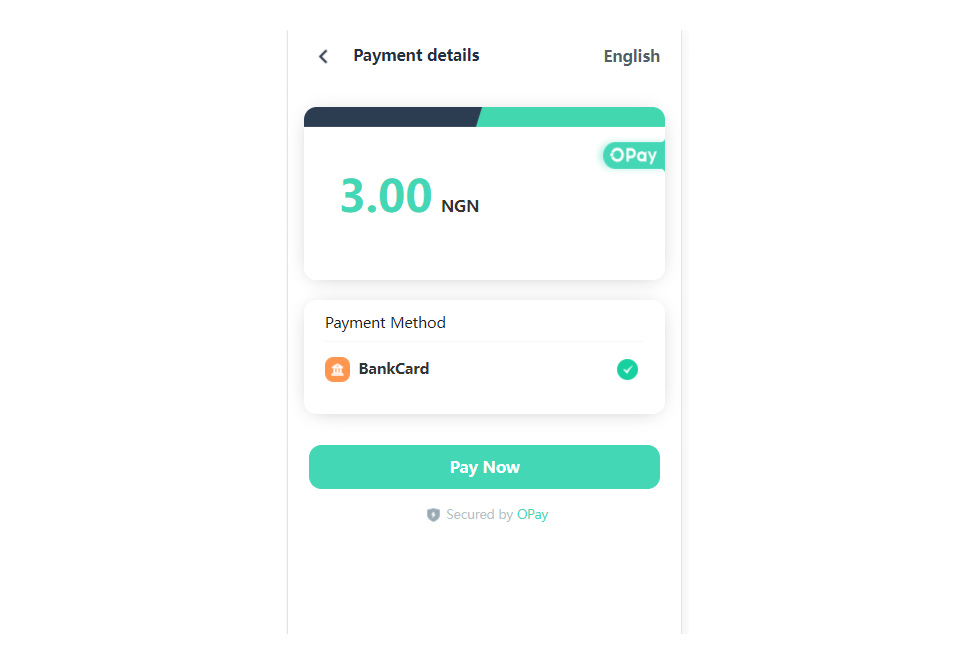
If you've left the payMethod
key blank in your request, your client will have the options to pay using:
- MasterCard, VISA, or MEEZA cards.
- Obtain a reference number and pay at any POS of our OPay retail network.
Otherwise, if you have designated a specific payMethod
, only the designated payment method will be available to your client to use.
Payment Processing Information
If your client has completed the payment at the generated cashierUrl
,
he will be redirected to the returnUrl
sent with your request, otherwise,
if he decided to cancel the payment, he will be redirected to cancelUrl
.
You will receive the payment processing information on the callbackUrl
provided with your API request.
For more information regarding handling callbacks, please refer to the Payment Notifications Callback section.
Error Handling
After submitting an API call to OPay, you receive a response back to inform you that
your request was received and processed. A successful OPay API should return a status code 00000
,
meanwhile, in a situation where any payment processing error occurred, you will receive an error code with a
message to describe the reason of the error. A sample error response can be found below.
{
"code": "02004",
"message": "the payment reference already exists."
}
Depending on the HTTP status code of the response, you should build some logic to handle any errors that a request or the system may return. A list of possible potential error codes that you may receive can be found below.
Error Code | Error Message |
---|---|
02000 | authentication failed. |
02001 | request params not valid. |
02003 | payMethod not support. |
02004 | the payment reference(merchant order number) already exists. |
02002 | merchant not configured with this function. |
02007 | merchant not available. |
50003 | service not available, please try again. |