OPay React Native
SDK
All payment solutions offered by OPay readily available in your clients' hands.
Prerequisites
OPay SDK will allow your React Native
application to:
- Launch full-screen activities to collect payment details, shipping address, and shipping method.
- Accept card, reference code, installment payment readily available from OPay.
Make sure you have an active OPay merchant account, or create account.
To avoid any conflicts and have a seamless integration, make sure you have the following compatibility list satisfied.
ITEM | COMPATIBLE VERSION | REFERENCES |
---|---|---|
react-native-webview |
>=8.0.0 | |
react-native |
>=0.51 | |
crypto-js |
>=3.2.0 |
How React Native
SDK Plugin Looks Like
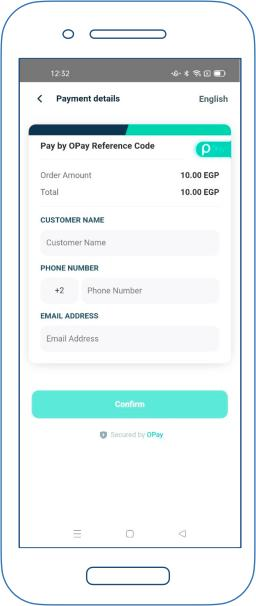
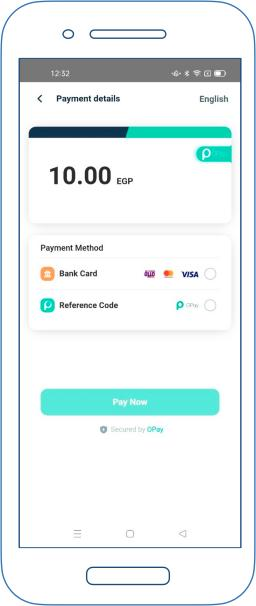
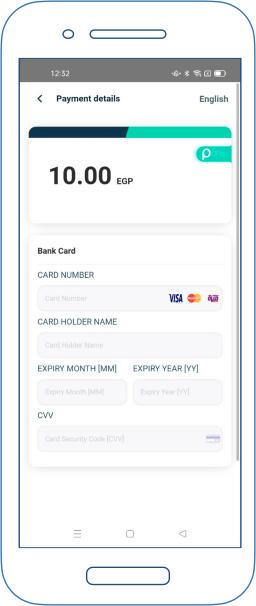
How it works
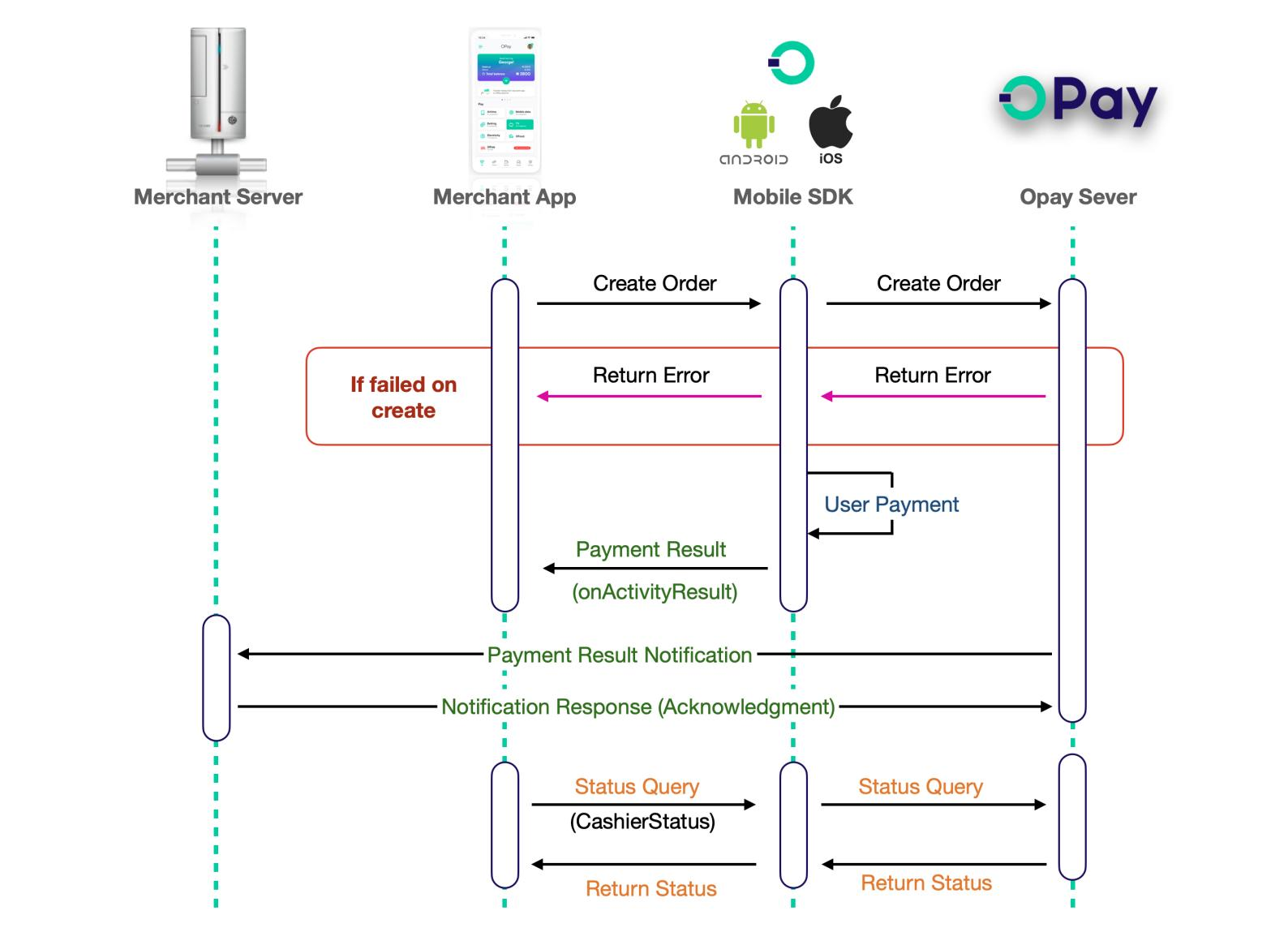
On this page we will walk you through React Native
SDK integration steps:
- Initialize and configure
React Native
SDK. - Present Payment options and collect you client's payment data.
- OPay
React Native
SDK plugin will send payment request to OPay. - Return payment processing information to our
React Native
SDK plugin. - Within your
React Native
application, inform your client with the payment result.
SDK Integration
Please follow the below steps in order to get the SDK integrated and ready for use within your application logic.
-
need to remove the old version first if you already have it,run:
npm uninstall opay-online-rn-sdk yarn remove opay-online-rn-sdk
-
in your project directory,run:
npm: npm install opay-online-payment-rn-sdk yarn: yarn add opay-online-payment-rn-sdk
-
Installing dependencies into a bare React Native project:
npm: npm install react-native-webview yarn: yarn add react-native-webview
If your React Native is 0.59 and lower,you need to linking.react-native link react-native-webview
From React Native 0.60 and higher, linking is automatic.
If you're on a Mac and developing for iOS, you need to install the pods (via Cocoapods) to complete the linking.cd ios pod install
SDK API Hooks
After integrating OPay SDK, now it's time to know how to use our SDK API hooks to:
- Toggle between isSandBox and Production environment.
- Create payment order.
- Handling payment result.
- Order status query.
Setup SDK Environment
During the development and integration phase, you may use our SDK in testing mode be setting the isSandBox to true:
OPay.isSandBox=true
Once you are done with your testing and your application is now ready for production, you can toggle false the isSandBox:
OPay.isSandBox=false
Create Payment Order
Add OPayPage(A Component)
into react-navigation route config.
Your app cart item should implement PayParams
object, the below table
illustrates the values that should be passed through your PayParams
object.
Parameter | type | Required | Description | |
---|---|---|---|---|
publicKey | String |
required | Your OPay merchant account public key. | |
merchantId | String |
required | Your OPay account merchant ID. | |
merchantName | String |
required | Merchant Name to be displayed in cashier checkout form. | |
reference | String |
required | Payment reference number in your system. | |
countryCode | String |
required | Country code (NG). | |
currency | String |
required | Currency type (NGN). | |
payAmount | Long |
required | payment total amount. | |
productName | String |
required | Name of Product/Service to be purchased. | |
productDescription | String |
required | Description of Product/Service to be purchased. | |
callbackUrl | String |
optional | If you have sent callbackUrl through API, OPay will send callback notification to this callbackUrl. If you didn't send callbackUrl through API, you need to configure webhook url on the merchant dashboard, and OPay will send callback notification to this webhook url. See callback here | |
expireAt | Integer |
required | Payment expiration time in minutes. | |
payMethod | String |
optional | The preferred payment method to be presented to your customer. See available options here. If not set, all supported payment methods will be available to your customer to choose from. | |
userInfo JSON Object |
||||
userId | String |
optional | the customer user id | |
userName | String |
optional | the customer user name | |
userMobile | String |
optional | the customer user mobile | |
userEmail | String |
optional | the customer user email |
Create PayInput PayParams
object is given below.
let payInput = {
publicKey : "{PublicKey}",// your public key
merchantId : "256612345678901",// your merchant id
merchantName : "TEST 123",
reference : "12347544444555666",// reference unique, must be updated on each request
countryCode : "NG", // uppercase
currency : "NGN", // uppercase
payAmount : 10000,
productName : "",
productDescription :"",
callbackUrl :"http://www.callbackurl.com",
userClientIP :"110.246.160.183",
expireAt :30,
paymentType :"", // optional
// optional
userInfo = {
userId:"userId",
userEmail:"userEmail",
userMobile:"userMobile",
userName:"uesrName"
}
}
After initiating the required PayParams data, you need to call props.navigation.push() to start OPayPage to ceate order. Code example as follows:
this.props.navigation.push("OPayPageRouteName", // your defined route name
{
payParams:this.payParams,
httpCallback:this.orderHttpCallback,
webPayCallback:this.orderWebPayCallback,
});
/**
* http callback from order api
* @param response
*/
orderHttpCallback=(response)=>{
console.log(JSON.stringify(response))
}
/**
* web callback from pay page than behind order api
* @param webJsResponse
*/
orderWebPayCallback=(webJsResponse:OPayWebJsResponse)=>{
if(webJsResponse!=null){
let status = webJsResponse.orderStatus;
console.log(JSON.stringify(webJsResponse))
switch(status){
case PayResultStatus.initial:
break;
case PayResultStatus.pending:
break;
case PayResultStatus.success:
break;
case PayResultStatus.fail:
break;
case PayResultStatus.close:
break;
}
}
}
Handling Payment Result
Once the payment has been completed by your client, OPay server will respond back to the SDK to let you know the payment processing result. After your client completes the payment, you will get the payment processing result in the form of Json object. :
Sample response Json
object.
{
"callbackName": "clickReferenceCodeReturnBtn",
"eventName": "clickReferenceCodeReturnBtn",
"merchantId": "256612345678901",
"orderNo": "211026140930379662",
"orderStatus": "PENDING", // INITIAL - SUCCESS - FAIL - CLOSE
"payNo": "211026140930379662",
"referenceCode": "607943772"
}
Due to network and any other issues, the order status may not be timely. It is highly recommended to call order status inquiry to ensure the accuracy.
Order Query Status
Due to network and any other issues, the order status may not be timely. It is highly recommended
to call order status inquiry to ensure the integrity of your data. To that end,
You will need to create a CashierStatusParams
object that contains the following request parameters:
Parameter | type | Required | Description |
---|---|---|---|
publicKey | String |
required | Your OPay merchant account public key. |
merchantId | String |
required | Your OPay account merchant ID. |
reference | String |
required | Payment reference number in your system. |
countryCode | String |
required | Country code (NG). |
let cashierStatusParams ={
privateKey:"OPAYPRV16387746262010.7102038091157474",
merchantId:"281821120675251",
reference:"123",
countryCode : OPayCountry.NIGERIA.countryCode
}
After creating the cashierStatusParams
object, you need to call the new OPay().getCashierStatus(params)
method to query order status and process the subsequent logic according to the response object returned.
new OPay().getCashierStatus(cashierStatusParam).then((response){
let status = response.data.status
switch(status){
case PayResultStatus.initial:
break;
case PayResultStatus.pending:
break;
case PayResultStatus.success:
break;
case PayResultStatus.fail:
break;
case PayResultStatus.close:
break;
}});
Response data format is as follows:
{
"code": "00000",
"message": "SUCCESSFUL",
"data":{
"reference":"1001000",
"orderNo":"10212100000034000",
"status":"SUCCESS",
"vat":{
"total":10000,
"currency":"NGN",
"rate":100,
"currencySymbo":"₦"
},
"failureReason":null,
"silence":"Y"
}
}